Programmable logic in PHP
What is it?
Parallel ROMs (OTP-ROMs, EPROMs, EEPROMs, NOR flash, etc.) can be used as simple, programmable logic devices.
They can be programmed to hold a lookup-table / bit stream, which will emulate logic functions (like 74-series logic) by responding to a certain pattern on the “input” (aka address lines) with a pre-calculated “output” (on the data lines).
Generating the bit patterns to flash onto such an EEPROM can be tedious and error-prone, when done by hand (with a hex-editor).
PHPPLD provides a simple and easy way to generate complex logic as a bit stream. The user can provide a small function, written in the PHP programming language. This function is then called for each possible combination of bits on the EEPROM’s “input”/address-lines. For a 128KiB flash ROM (like a MX29F001B), this means the function will get called 131072 times.
How does it work?
The PHP function can react to the input bits in various ways.
They can be used as booleans (provided as an $input
array) and combined with logical functions:
// NOT gate
$output[0] = !$input[0];
// AND gate
$output[1] = $input[1] && $input[2];
It can also convert the input into a number (useful when connected to a microprocessor bus) and work as an address decoder:
$address = integer_value($input);
if ($address >= 0x20 && $address <= 0x40)
{
$cs_soundchip = 1;
}
if ($address >= 0x60 && $address <= 0x65)
{
$cs_videochip = 1;
}
$output[0] = $cs_soundchip;
$output[1] = !$cs_videochip; // Note the inversion for active-low signals
VGA signal generation
A rather extreme example of PHPPLD is the vga.php.
It uses a binary counter connected to the address inputs of the EEPROM. This counter is being fed from an external clock.
The output bits of the EEPROM are:
- 2 bits of red color information
- 2 bits of green color information
- 1 bit of blue color information
- pattern finish bit / counter reset
- horizontal sync
- vertical sync
While evaluating the script, PHPPLD can use GDlib (an image manipulation library) to look at individual pixels of an image file and store the appropriate color values into the bit stream. It will also calculate the timing information/sync signals required for a VGA monitor.
This generates a fixed 800x600 signal, with an effective resolution of 100x600 pixels.
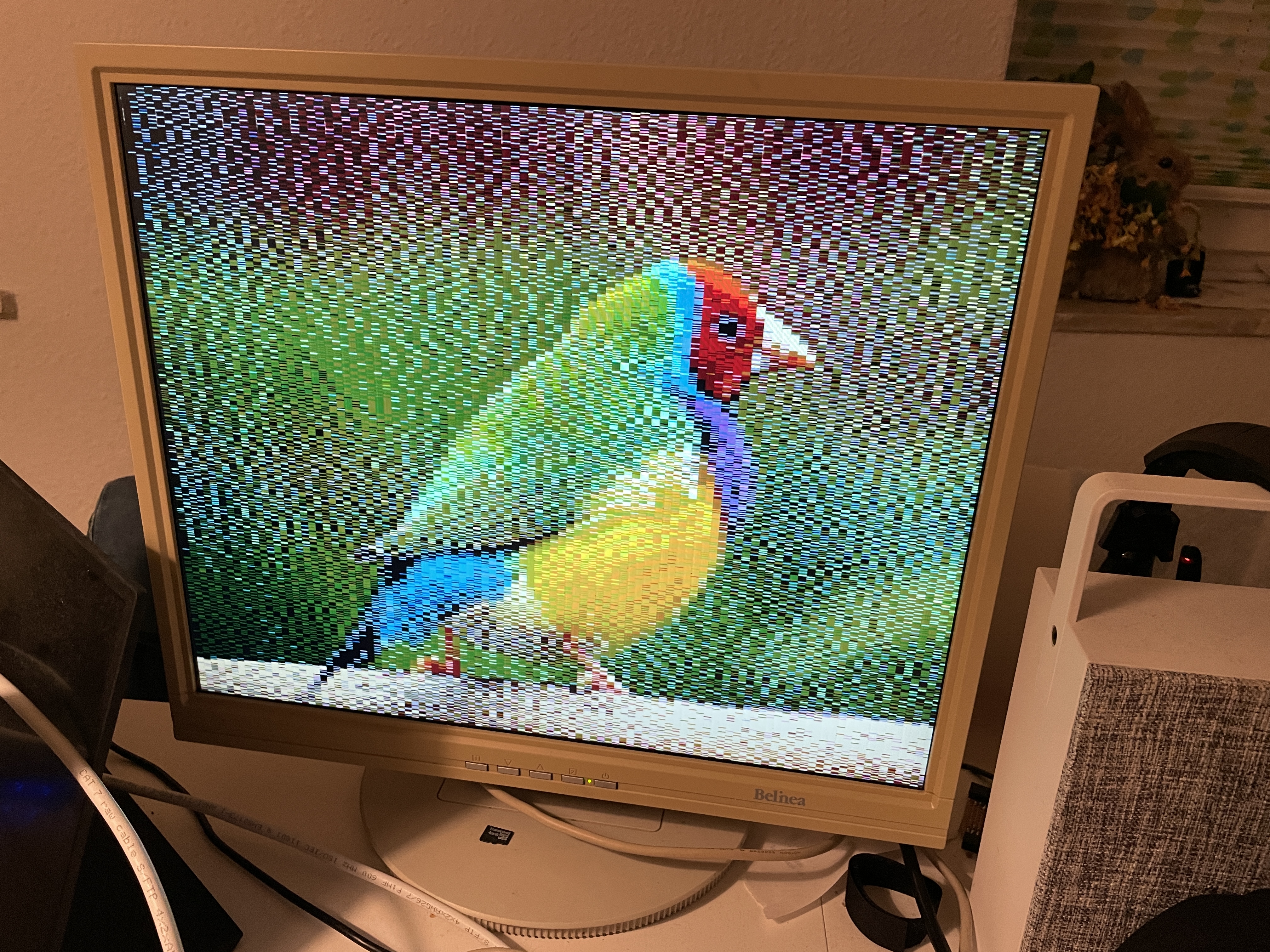
ISA bus decoder
PHPPLD was used to generate a bitstream to map a RC2014 (Z80-based computer) extension card onto a x86-PC ISA bus.
This allowed the use of the RTL8019 network chip on the RC2014 card on a regular PC before the ISA8019 card was finished.